Loading...
Searching...
No Matches
Scenario::IntervalModel Class Referencefinal
Inheritance diagram for Scenario::IntervalModel:
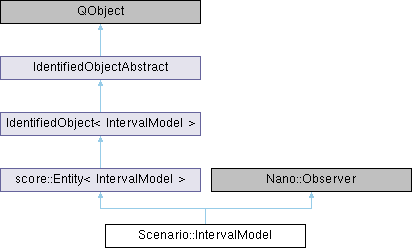
Public Types | |
enum | ViewMode : bool { Temporal = 0 , Nodal = 1 } |
![]() | |
using | entity_type = Entity< IntervalModel > |
![]() | |
using | model_type = model |
using | id_type = Id< model > |
using | object_type = IdentifiedObject< model > |
Public Member Functions | |
IntervalModel (const Id< IntervalModel > &, double yPos, const score::DocumentContext &ctx, QObject *parent) | |
IntervalModel (DataStream::Deserializer &vis, const score::DocumentContext &ctx, QObject *parent) | |
IntervalModel (JSONObject::Deserializer &vis, const score::DocumentContext &ctx, QObject *parent) | |
IntervalModel (DataStream::Deserializer &&vis, const score::DocumentContext &ctx, QObject *parent) | |
IntervalModel (JSONObject::Deserializer &&vis, const score::DocumentContext &ctx, QObject *parent) | |
const score::DocumentContext & | context () const noexcept |
const Id< StateModel > & | startState () const noexcept |
void | setStartState (const Id< StateModel > &eventId) |
const Id< StateModel > & | endState () const noexcept |
void | setEndState (const Id< StateModel > &endState) |
const TimeVal & | date () const noexcept |
void | setStartDate (const TimeVal &start) |
void | translate (const TimeVal &deltaTime) |
double | heightPercentage () const noexcept |
void | startExecution () |
void | stopExecution () |
void | reset () |
void | setHeightPercentage (double arg) |
void | setExecutionState (IntervalExecutionState) |
IntervalExecutionState | executionState () const |
ViewMode | viewMode () const noexcept |
void | setViewMode (ViewMode v) |
void | viewModeChanged (ViewMode v) |
QPointF | nodalOffset () const noexcept |
void | setNodalOffset (QPointF offs) |
double | nodalScale () const noexcept |
void | setNodalScale (double z) |
ZoomRatio | zoom () const noexcept |
void | setZoom (const ::ZoomRatio &zoom) |
TimeVal | midTime () const noexcept |
void | setMidTime (const TimeVal &value) |
void | setSmallViewVisible (bool) |
bool | smallViewVisible () const noexcept |
const Rack & | smallView () const noexcept |
const FullRack & | fullView () const noexcept |
void | clearSmallView () |
void | clearFullView () |
void | replaceSmallView (const Rack &other) |
void | replaceFullView (const FullRack &other) |
void | addSlot (Slot s) |
void | addSlot (Slot s, int pos) |
void | removeSlot (int pos) |
void | addLayer (int slot, Id< Process::ProcessModel >) |
void | removeLayer (int slot, Id< Process::ProcessModel >) |
void | putLayerToFront (int slot, Id< Process::ProcessModel >) |
void | putLayerToFront (int slot, std::nullopt_t) |
void | swapSlots (int pos1, int pos2, Slot::RackView fullview) |
double | getSlotHeight (const SlotId &slot) const |
void | setSlotHeight (const SlotId &slot, double height) |
double | getHeight () const noexcept |
double | getSlotHeightForProcess (const Id< Process::ProcessModel > &p) const |
Must be called with a process which is a child of this interval. | |
const Slot * | findSmallViewSlot (int slot) const |
const Slot & | getSmallViewSlot (int slot) const |
Slot & | getSmallViewSlot (int slot) |
const FullSlot * | findFullViewSlot (int slot) const |
const FullSlot & | getFullViewSlot (int slot) const |
FullSlot & | getFullViewSlot (int slot) |
bool | muted () const noexcept |
void | setMuted (bool m) |
bool | graphal () const noexcept |
void | setGraphal (bool m) |
bool | executing () const noexcept |
void | setExecuting (bool m) |
bool | hasTimeSignature () const noexcept |
void | setHasTimeSignature (bool b) |
TimeVal | contentDuration () const noexcept |
TempoProcess * | tempoCurve () const noexcept |
void | ancestorStartDateChanged () |
void | ancestorTempoChanged () |
void | addSignature (TimeVal t, ossia::time_signature sig) |
void | removeSignature (TimeVal t) |
void | setTimeSignatureMap (const TimeSignatureMap &map) |
const TimeSignatureMap & | timeSignatureMap () const noexcept |
ossia::musical_sync | quantizationRate () const noexcept |
void | setQuantizationRate (ossia::musical_sync b) |
void | setStartMarker (TimeVal t) |
TimeVal | startMarker () const noexcept |
void | hasTimeSignatureChanged (bool arg_1) |
void | timeSignaturesChanged (const TimeSignatureMap &arg_1) |
void | quantizationRateChanged (ossia::musical_sync arg) |
void | requestHeightChange (double y) |
void | heightPercentageChanged (double arg_1) |
void | dateChanged (const TimeVal &arg_1) |
void | startMarkerChanged (const TimeVal &arg_1) |
void | focusChanged (bool arg_1) |
void | executionStateChanged (Scenario::IntervalExecutionState arg_1) |
void | executionEvent (Scenario::IntervalExecutionEvent ev) |
void | smallViewVisibleChanged (bool fv) |
void | rackChanged (Scenario::Slot::RackView fv) |
void | slotAdded (Scenario::SlotId arg_1) |
void | slotRemoved (Scenario::SlotId arg_1) |
void | slotResized (Scenario::SlotId arg_1) |
void | slotsSwapped (int slot1, int slot2, Slot::RackView fv) |
void | heightFinishedChanging () |
void | layerAdded (Scenario::SlotId arg_1, Id< Process::ProcessModel > arg_2) |
void | layerRemoved (Scenario::SlotId arg_1, Id< Process::ProcessModel > arg_2) |
void | frontLayerChanged (int arg_1, OptionalId< Process::ProcessModel > arg_2) |
void | mutedChanged (bool arg_1) |
void | executingChanged (bool arg_1) |
void | busChanged (bool arg_1) |
void | graphalChanged (bool arg_1) |
void | nodalOffsetChanged (QPointF arg_1) |
void | nodalScaleChanged (double arg_1) |
![]() | |
Entity (Id< IntervalModel > id, const QString &name, QObject *parent) noexcept | |
Entity (Visitor &&vis, QObject *parent) noexcept | |
const score::Components & | components () const noexcept |
score::Components & | components () noexcept |
const score::ModelMetadata & | metadata () const noexcept |
score::ModelMetadata & | metadata () noexcept |
![]() | |
IdentifiedObject (id_type id, const QString &name, QObject *parent) noexcept | |
template<typename Visitor > | |
IdentifiedObject (Visitor &&v, QObject *parent) noexcept | |
const id_type & | id () const noexcept |
int32_t | id_val () const noexcept final override |
void | setId (const id_type &id) noexcept |
void | setId (id_type &&id) noexcept |
void | resetCache () const noexcept override |
![]() | |
void | identified_object_destroying (IdentifiedObjectAbstract *o) |
To be called by subclasses. | |
void | identified_object_destroyed (IdentifiedObjectAbstract *o) |
Will be called in the IdentifiedObjectAbstract destructor. | |
Public Attributes | |
std::unique_ptr< Process::AudioInlet > | inlet |
std::unique_ptr< Process::AudioOutlet > | outlet |
score::EntityMap< Process::ProcessModel, true > | processes |
Selectable | selection {this} |
ModelConsistency | consistency {nullptr} |
IntervalDurations | duration {*this} |
![]() | |
Path< model > | m_path_cache |
Friends | |
struct | IntervalSaveData |
struct | SlotPath |
Additional Inherited Members | |
![]() | |
static const constexpr bool | entity_tag |
![]() | |
static const constexpr bool | identified_object_tag = true |
![]() | |
IdentifiedObjectAbstract (const QString &name, QObject *parent) noexcept | |
Constructor & Destructor Documentation
◆ IntervalModel()
Scenario::IntervalModel::IntervalModel | ( | const Id< IntervalModel > & | id, |
double | yPos, | ||
const score::DocumentContext & | ctx, | ||
QObject * | parent | ||
) |
The class
Member Data Documentation
◆ processes
score::EntityMap<Process::ProcessModel, true> Scenario::IntervalModel::processes |
Properties of the class
The documentation for this class was generated from the following files:
- IntervalModel.hpp
- IntervalModel.cpp